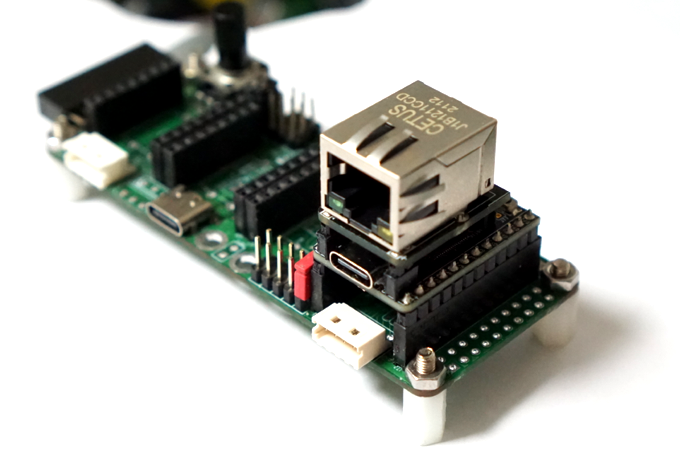
기존의 RP2040과 W5500을 테스트 했던 Arduino 개발환경 에서는 DCHP가 자동으로 설정되지만 SDK를 이용하면 직접 코드를 작성해야 한다. 아쉽다... 이걸 다시 작성해야 하다니..
아무튼 DHCP 예제를 참고로 해서 테스트 해보자.
#include "port_common.h"
#include "wizchip_conf.h"
#include "w5x00_spi.h"
#include "socket.h"
#include "dhcp/dhcp.h"
#include "timer/timer.h"
/* Buffer */
#define ETHERNET_BUF_MAX_SIZE (1024 * 4)
#define DATA_BUF_SIZE ETHERNET_BUF_MAX_SIZE
/* Socket */
#define SOCKET_DHCP 0
#define SOCKET_DNS 1
#define DHCP_RETRY_COUNT 5
/* Port */
#define PORT_LOOPBACK 5001
/**
* ----------------------------------------------------------------------------------------------------
* Variables
* ----------------------------------------------------------------------------------------------------
*/
/* Network */
static wiz_NetInfo g_net_info =
{
.mac = {0x00, 0x08, 0xDC, 0x00, 0x00, 0x00}, // MAC address
.ip = {192, 168, 1, 4}, // IP address
.sn = {255, 255, 255, 0}, // Subnet Mask
.gw = {192, 168, 1, 1}, // Gateway
.dns = {8, 8, 8, 8}, // DNS server
.dhcp = NETINFO_DHCP // DHCP enable/disable
};
/* Loopback */
static uint8_t g_ethernet_buf[ETHERNET_BUF_MAX_SIZE] = {
0,
}; // common buffer
/* DHCP */
static uint8_t g_dhcp_get_ip_flag = 0;
/* Timer */
static volatile uint16_t g_msec_cnt = 0;
/**
* ----------------------------------------------------------------------------------------------------
* Functions
* ----------------------------------------------------------------------------------------------------
*/
/* Clock */
static void set_clock_khz(void);
/* DHCP */
static void wizchip_dhcp_init(void);
static void wizchip_dhcp_assign(void);
static void wizchip_dhcp_conflict(void);
/* Timer */
static void repeating_timer_callback(void);
int main()
{
uint8_t retval = 0;
uint8_t dhcp_retry = 0;
uint8_t dns_retry = 0;
int cnt = 0;
stdio_init_all();
while (true) {
if(cnt>5)break;
printf("%d]Hello, world!\n", cnt++);
sleep_ms(1000);
}
// For more examples of UART use see https://github.com/raspberrypi/pico-examples/tree/master/uart
printf("W55RP20 Start\n");
wizchip_spi_initialize();
wizchip_cris_initialize();
wizchip_reset();
printf("wizchip_initialize");
wizchip_initialize();
wizchip_check();
/* Get network information */
//print_network_information(g_net_info);
wizchip_1ms_timer_initialize(repeating_timer_callback);
if (g_net_info.dhcp == NETINFO_DHCP) // DHCP
{
wizchip_dhcp_init();
}
else // static
{
network_initialize(g_net_info);
/* Get network information */
print_network_information(g_net_info);
}
while (true) {
/* Assigned IP through DHCP */
if (g_net_info.dhcp == NETINFO_DHCP)
{
retval = DHCP_run();
if (retval == DHCP_IP_LEASED)
{
if (g_dhcp_get_ip_flag == 0)
{
printf(" DHCP success\n");
g_dhcp_get_ip_flag = 1;
}
}
else if (retval == DHCP_FAILED)
{
g_dhcp_get_ip_flag = 0;
dhcp_retry++;
if (dhcp_retry <= DHCP_RETRY_COUNT)
{
printf(" DHCP timeout occurred and retry %d\n", dhcp_retry);
}
}
if (dhcp_retry > DHCP_RETRY_COUNT)
{
printf(" DHCP failed\n");
DHCP_stop();
while (1)
;
}
wizchip_delay_ms(1000); // wait for 1 second
}
}
}
/* DHCP */
static void wizchip_dhcp_init(void)
{
printf(" DHCP client running\n");
DHCP_init(SOCKET_DHCP, g_ethernet_buf);
reg_dhcp_cbfunc(wizchip_dhcp_assign, wizchip_dhcp_assign, wizchip_dhcp_conflict);
}
static void wizchip_dhcp_assign(void)
{
getIPfromDHCP(g_net_info.ip);
getGWfromDHCP(g_net_info.gw);
getSNfromDHCP(g_net_info.sn);
getDNSfromDHCP(g_net_info.dns);
g_net_info.dhcp = NETINFO_DHCP;
/* Network initialize */
network_initialize(g_net_info); // apply from DHCP
print_network_information(g_net_info);
printf(" DHCP leased time : %ld seconds\n", getDHCPLeasetime());
}
static void wizchip_dhcp_conflict(void)
{
printf(" Conflict IP from DHCP\n");
// halt or reset or any...
while (1)
; // this example is halt.
}
/* Timer */
static void repeating_timer_callback(void)
{
g_msec_cnt++;
if (g_msec_cnt >= 1000 - 1)
{
g_msec_cnt = 0;
DHCP_time_handler();
}
}
테스트 결과 DHCP로 IP를 정상적으로 할당 받는것을 확인 할 수 있다.
wizchip_check -> OK
DHCP client running
============================================================================================
W5500 network configuration : DHCP
MAC : 00:08:DC:00:00:00
IP : 192.168.1.6
Subnet Mask : 255.255.255.0
Gateway : 192.168.1.1
DNS :
============================================================================================
DHCP leased time : 7200 seconds
DHCP success
반응형