ESP32-C3를 이용하여 BLE UART를 테스트 해보자
기본 펌웨어 다운로드 하고 장치 검색하면 설정한 장치로 인식이 된다
// Create the BLE Device
BLEDevice::init("ECPC3 UART");
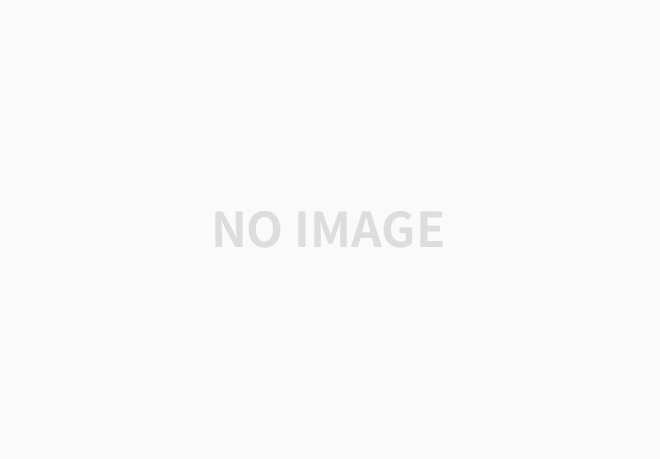
BLE UART 초기화
#define SERVICE_UUID "6E400001-B5A3-F393-E0A9-E50E24DCCA9E" // UART service UUID
#define CHARACTERISTIC_UUID_RX "6E400002-B5A3-F393-E0A9-E50E24DCCA9E"
#define CHARACTERISTIC_UUID_TX "6E400003-B5A3-F393-E0A9-E50E24DCCA9E"
//1)BLE Device를 생성하고
BLEDevice::init("ECPC3 UART");
//2)BLE Server 생성 하고
pServer = BLEDevice::createServer();
pServer->setCallbacks(new MyServerCallbacks());
//3)BLE Service 생성하고
BLEService *pService = pServer->createService(SERVICE_UUID);
//4)BLE Characteristic생성하고 등록 한다.
pTxCharacteristic = pService->createCharacteristic(
CHARACTERISTIC_UUID_TX,
BLECharacteristic::PROPERTY_NOTIFY
);
pTxCharacteristic->addDescriptor(new BLE2902());
BLECharacteristic * pRxCharacteristic = pService->createCharacteristic(
CHARACTERISTIC_UUID_RX,
BLECharacteristic::PROPERTY_WRITE
);
pRxCharacteristic->setCallbacks(new MyCallbacks());
//5)Service 시작
pService->start();
//6)advertising - BLE 검색 시작
pServer->getAdvertising()->start();
Serial.println("Waiting a client connection to notify...");
앱은 기존에 사용 했던 nRF Toolbox 를 사용 했다.
접속되고 데이터 전송이 된다.
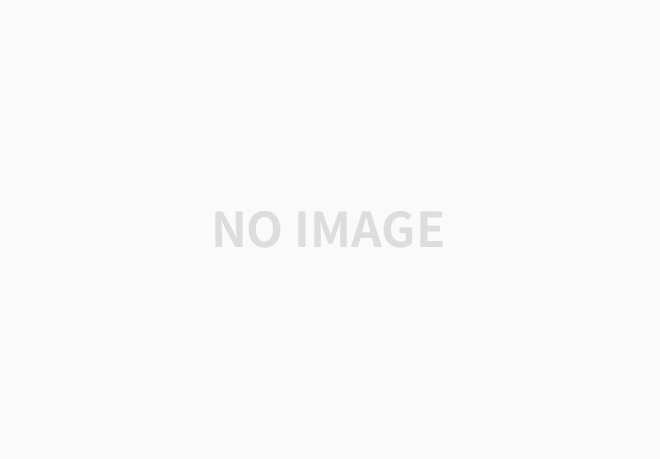
하지만 또 문제가 생겼다.
전송은 되지만 상당히 느리다.
코드를 자세히 보니 10ms마다 데이터를 송신하도록 되어 있는데 이부분을 주석 처리 하니 잘 동작한다.
void loop() {
if (deviceConnected)
{
//pTxCharacteristic->setValue(&txValue, 1);
//pTxCharacteristic->notify();
//txValue++;
//delay(10); // bluetooth stack will go into congestion, if too many packets are sent
digitalWrite(LED_PIN2, 0);
}
간단히 키값을 등록해서 ESP32-C3 SSM 보드의 LED를 제어 할 수 있도록 해 보았다.
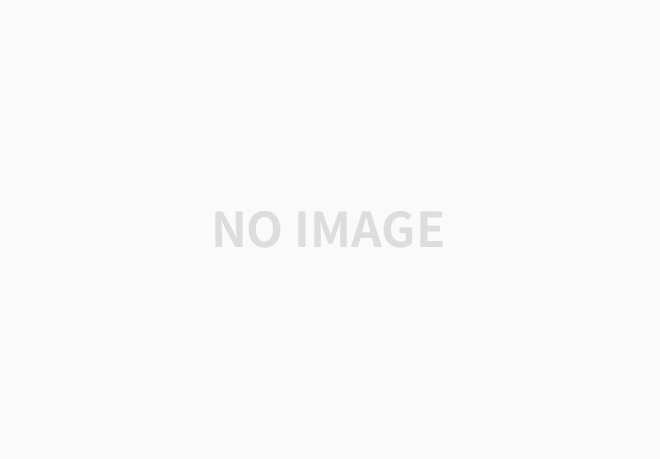
ESP32-C3 BLE UART 테스트 코드
#include <BLEDevice.h>
#include <BLEServer.h>
#include <BLEUtils.h>
#include <BLE2902.h>
#define LED_PIN1 3
#define LED_PIN2 7
BLEServer *pServer = NULL;
BLECharacteristic * pTxCharacteristic;
bool deviceConnected = false;
bool oldDeviceConnected = false;
uint8_t txValue = 0;
// See the following for generating UUIDs:
// https://www.uuidgenerator.net/
#define SERVICE_UUID "6E400001-B5A3-F393-E0A9-E50E24DCCA9E" // UART service UUID
#define CHARACTERISTIC_UUID_RX "6E400002-B5A3-F393-E0A9-E50E24DCCA9E"
#define CHARACTERISTIC_UUID_TX "6E400003-B5A3-F393-E0A9-E50E24DCCA9E"
class MyServerCallbacks: public BLEServerCallbacks {
void onConnect(BLEServer* pServer) {
deviceConnected = true;
};
void onDisconnect(BLEServer* pServer) {
deviceConnected = false;
}
};
class MyCallbacks: public BLECharacteristicCallbacks {
void onWrite(BLECharacteristic *pCharacteristic) {
std::string rxValue = pCharacteristic->getValue();
if (rxValue.length() > 0) {
//Serial.println("*********");
Serial.print("Received Value: ");
for (int i = 0; i < rxValue.length(); i++)
Serial.print(rxValue[i]);
Serial.println();
if(rxValue[0] == 'a')
digitalWrite(LED_PIN1, 0);
else if(rxValue[0] == 'b')
digitalWrite(LED_PIN1, 1);
//Serial.println("*********");
}
}
};
void setup() {
Serial.begin(115200);
pinMode(LED_PIN1, OUTPUT);
pinMode(LED_PIN2, OUTPUT);
digitalWrite(LED_PIN1, 1);
digitalWrite(LED_PIN2, 1);
//1)Create the BLE Device
BLEDevice::init("ECPC3 UART");
//2)Create the BLE Server
pServer = BLEDevice::createServer();
pServer->setCallbacks(new MyServerCallbacks());
//3)Create the BLE Service
BLEService *pService = pServer->createService(SERVICE_UUID);
//4)Create a BLE Characteristic
pTxCharacteristic = pService->createCharacteristic(
CHARACTERISTIC_UUID_TX,
BLECharacteristic::PROPERTY_NOTIFY
);
pTxCharacteristic->addDescriptor(new BLE2902());
BLECharacteristic * pRxCharacteristic = pService->createCharacteristic(
CHARACTERISTIC_UUID_RX,
BLECharacteristic::PROPERTY_WRITE
);
pRxCharacteristic->setCallbacks(new MyCallbacks());
//6)Start the service
pService->start();
//7)Start advertising
pServer->getAdvertising()->start();
Serial.println("Waiting a client connection to notify...");
}
void loop() {
if (deviceConnected)
{
//delay(10); // bluetooth stack will go into congestion, if too many packets are sent
digitalWrite(LED_PIN2, 0);
}
else
{
digitalWrite(LED_PIN2, 1);
}
// disconnecting
if (!deviceConnected && oldDeviceConnected) {
delay(500); // give the bluetooth stack the chance to get things ready
pServer->startAdvertising(); // restart advertising
Serial.println("start advertising");
oldDeviceConnected = deviceConnected;
}
// connecting
if (deviceConnected && !oldDeviceConnected) {
// do stuff here on connecting
oldDeviceConnected = deviceConnected;
}
}
반응형